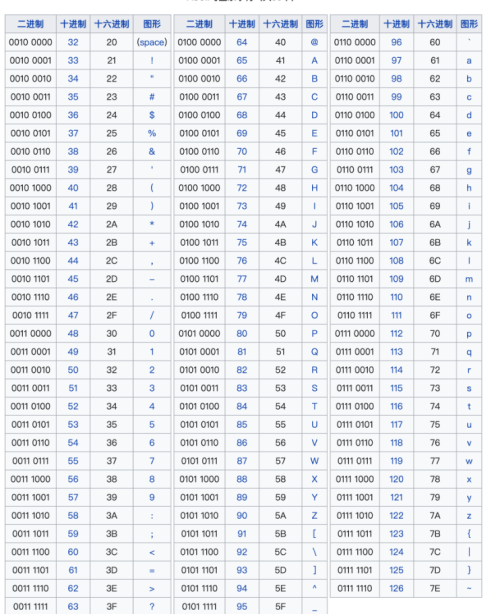
C语言中的字符串库函数
C语言字符串库函数
在本文中,我们将探讨一些常用的 C 语言字符串库函数,包括 strlen()
, strcpy()
, strncpy()
, strcat()
, strncat()
和 strcmp()
。对于每个函数,我们将展示其用法,并提供一个自定义的实现。
1. strlen()
strlen()
函数接受一个字符串作为参数,并返回字符串的长度。请注意,它不计算结尾的 \0
。
1 | strlen("ABC") = 3 |
下面是一个常用的计算字符串长度的方法:
1 | const char* p = s; |
2. strcpy() & strncpy()
接下来我们来看 strcpy()
和 strncpy()
函数。这两个函数用于复制字符串。
首先是 strcpy()
函数的使用:
1 | char s1[MAXLINE]; |
然后是 strncpy()
函数的使用:
1 | char s2[MAXLINE]; |
下面是 strcpy()
和 strncpy()
的自定义实现:
1 | char* my_strcpy(char* s1, const char* s2) { |
3. strcat() & strncat()
接下来我们来看 strcat()
和 strncat()
函数。这两个函数用于连接字符串。
首先是 strcat()
函数的使用:
1 | char s1[MAXLINE] = "Hello "; |
然后是 strncat()
函数的使用:
1 | char s2[MAXLINE] = "Hello "; |
下面是 strcat()
和 strncat()
的自定义实现:
1 | char* my_strcat(char* s1, const char* s2){ |
4. strcmp()
最后,我们来看 strcmp()
函数。这个函数用于比较两个字符串。
1 | printf("strcmp(%s, %s) = %d\n", "ABCDef", "ABCDe", strcmp("ABCDef", "ABCDe")); |
下面是 strcmp()
的自定义实现:
1 | int my_strcmp(const char* s1, const char* s2){ |
指针常量与常量指针
在学习字符串库函数的过程中,我们经常会遇到“指针常量”和“常量指针”这两个术语。这两个术语可能会引起混淆,特别是在它们的英文表述中,这两个概念的差异更为明显。让我们从英文的角度来解释这两个概念:
1. Pointer to const (指向常量的指针)
这种类型的指针可以指向不同的地址,但不能修改其指向地址处的数据。
1 | const int *ptr; // 指向整型常量的指针 |
2. Constant pointer (常量指针)
这种类型的指针一旦初始化后,其存储的地址就不能再被修改,但可以修改其指向地址处的数据。
1 | int value = 5; |
总结
- Pointer to const (“指向常量的指针”):允许改变指针指向的地址,但不允许通过指针修改所指向的数据。
- Constant pointer (“常量指针”):不允许改变指针的值(即地址),但允许修改指针指向地址处的数据。
通过这种方式理解,可以更清楚地区分这两种类型的指针,并理解它们在实际编程中的用途和限制。
- Title: C语言中的字符串库函数
- Author: van
- Created at : 2024-05-04 00:24:53
- Updated at : 2024-09-02 00:10:01
- Link: https://xblog.aptzone.cc/2024/05/04/C语言中的字符串库函数/
- License: All Rights Reserved © van
推荐阅读
推荐阅读
Comments